Java에서 난수 값을 생성하는 2가지 방법에 대해서 소개하려고 한다.
1-1. Math.random() : Math.random() 메서드는 0.0 ~ 1.0 사이의 값을 return 한다(Double값).
public class Task {
public static void main(String[] args){
for(int i=0; i<10; i++){
System.out.println(Math.random());
}
}
}
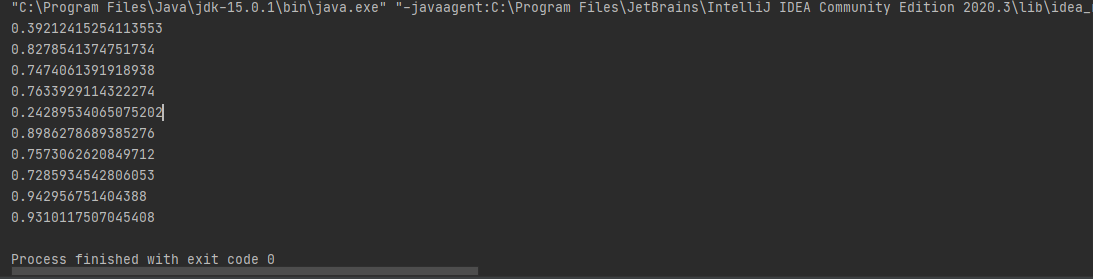
위 그림과 같이 10번을 print한 결과 0.0~1.0 사이의 값이 나오는 것을 볼 수 있다. 여기서 Int형으로 변환을 하고 싶을 때는 다음과 같이 하면 되는데, 주의사항이 한 가지 있다.
1-2. Math.random() Int형 변환 및 주의사항.
먼저 다음 코드 2개의 결과를 보도록 하자.
코드1.
public class Task {
public static void main(String[] args){
for(int i=0; i<10; i++){
System.out.println((int)(Math.random()*10));
}
}
}

코드 2.
public class Task {
public static void main(String[] args){
for(int i=0; i<10; i++){
System.out.println((int)Math.random()*10);
}
}
}

(코드 1)은 0~9까지의 값을 출력하는데, (코드 2)에서는 모두 0의 값이 나오고 있다. 두 코드의 차이는 괄호이다. (코드 1)에서는 Math.random()*10에 대해서 괄호를 통해 먼저 실행하고 Int형으로 변환했지만, (코드 2)에서는 해당 괄호를 제거해서 Int형으로 먼저 변환되고 나서 10을 곱한 결과가 나온 거다. 이유는 연산자 우선순위 때문인데, 그림으로 쉽게 설명을 하면 다음과 같다.

마지막으로 난수를 생성할 때 시작하는 숫자가 0이 아닌 1부터 시작하고 싶다면 위 코드에서 1을 더해주면 된다. 이렇게 하면 난수 값의 범위는 1~10까지로 맞춰진다.
public class Task {
public static void main(String[] args){
for(int i=0; i<10; i++){
System.out.println((int)(Math.random()*10)+1);
}
}
}
2. Random Class 사용
난수 값을 생성하는 다른 방법으로는 Random 클래스를 사용하는 것이다. Random 클래스 안에는 정수형 이외에도 Double, Float, Boolean 등등 다양한 타입의 랜덤 값을 생성할 수 있다. 주의사항으로는 Math.random()과는 다르게 java.util.Random Class를 import 시켜줘야 사용할 수 있다. 정수형 난수를 생성할 때는 Random.nextInt()의 인자로 정수 값을 넣어줘야 해당하는 정수값을 기준으로 난수 값의 범위가 설정되어 나오게 된다.
public class Task {
public static void main(String[] args){
Random random = new Random();
// Random Class 내부에 nextInt() 메서드가 2개 존재
// nextInt() / nextInt(int n)
System.out.println(random.nextInt()); // 2의 32승까지의 범위로 랜덤하게 값이 나옴(음수 포함).
System.out.println(random.nextInt(10)); // 0~9까지
System.out.println(random.nextInt(10)+1); // 1~10까지
System.out.println(random.nextDouble());
System.out.println(random.nextBoolean());
}
}
정리(Int형 기준)
1. Math.random()를 Int형으로 변환 시 괄호 범위에 주의해야 한다.
2. Random Class의 nextInt() 사용 시 인자 값을 넣어 반드시 범위를 설정해 주어야 한다.
'Java > Java Tip' 카테고리의 다른 글
인텔리제이(IntelliJ) 프로젝트에서 동시에 여러 Run 실행하는 방법 (2) | 2021.05.12 |
---|---|
[Java] Scanner를 통해 문자를 입력 받는 방법 : CharAt(String -> Char 변환) (0) | 2021.05.09 |
[Java] Swing JTextArea 화면 꽉차게 나타내는법(Resizable) (0) | 2021.05.07 |